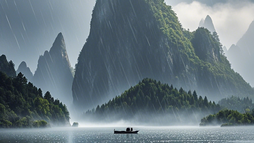
BP神经网络结合数据增强技术的应用
本文详细探讨了 BP 神经网络与数据增强技术相结合的应用。BP 神经网络作为一种强大的机器学习模型,在众多领域展现出其卓越的性能,但在处理数据时,尤其是当数据量有限或数据分布不均匀时,可能面临过拟合和泛化能力不足的问题。数据增强技术则为这些问题提供了有效的解决方案,通过扩充数据样本,引入更多的数据多样性,帮助 BP 神经网络更好地学习数据模式。本文将深入阐述 BP 神经网络的原理和数据增强技术的各
BP神经网络结合数据增强技术的应用
摘要: 本文详细探讨了 BP 神经网络与数据增强技术相结合的应用。BP 神经网络作为一种强大的机器学习模型,在众多领域展现出其卓越的性能,但在处理数据时,尤其是当数据量有限或数据分布不均匀时,可能面临过拟合和泛化能力不足的问题。数据增强技术则为这些问题提供了有效的解决方案,通过扩充数据样本,引入更多的数据多样性,帮助 BP 神经网络更好地学习数据模式。本文将深入阐述 BP 神经网络的原理和数据增强技术的各种方法,展示如何将两者结合应用于实际问题,并通过丰富的代码示例演示具体实现过程,为提高模型的性能和稳定性提供全面的指导。
一、BP 神经网络概述
(一)BP神经网络的基本原理
BP(Back Propagation)神经网络是一种多层前馈神经网络,其核心思想是通过正向传播将输入数据从输入层经过隐藏层处理,最终在输出层得到预测结果,然后根据预测结果与实际结果的误差,通过反向传播算法调整网络的权重和偏置,以最小化误差。
以下是一个简单的 BP 神经网络的 Python 代码示例,使用 numpy
实现:
import numpy as np
# 激活函数及其导数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(x):
return x * (1 - x)
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
# 初始化权重和偏置
self.weights_input_hidden = np.random.rand(input_size, hidden_size)
self.bias_hidden = np.random.rand(1, hidden_size)
self.weights_hidden_output = np.random.rand(hidden_size, output_size)
self.bias_output = np.random.rand(1, output_size)
def forward_propagation(self, inputs):
# 输入层到隐藏层
hidden_input = np.dot(inputs, self.weights_input_hidden) + self.bias_hidden
hidden_output = sigmoid(hidden_input)
# 隐藏层到输出层
output_input = np.dot(hidden_output, self.weights_hidden_output) + self.bias_output
output = sigmoid(output_input)
return hidden_output, output
def backward_propagation(self, inputs, hidden_output, output, expected):
# 计算输出层误差
output_error = expected - output
output_delta = output_error * sigmoid_derivative(output)
# 计算隐藏层误差
hidden_error = np.dot(output_delta, self.weights_hidden_output.T)
hidden_delta = hidden_error * sigmoid_derivative(hidden_output)
# 更新权重和偏置
self.weights_hidden_output += np.dot(hidden_output.T, output_delta)
self.bias_output += np.sum(output_delta, axis=0, keepdims=True)
self.weights_input_hidden += np.dot(inputs.T, hidden_delta)
self.bias_hidden += np.sum(hidden_delta, axis=0, keepdims=True)
def train(self, inputs, expected, epochs, learning_rate):
for epoch in range(epochs):
for i in range(len(inputs)):
hidden_output, output = self.forward_propagation(inputs[i].reshape(1, -1))
self.backward_propagation(inputs[i].reshape(1, -1), hidden_output, output, expected[i].reshape(1, -1))
# 示例使用
input_size = 2
hidden_size = 4
output_size = 1
nn = NeuralNetwork(input_size, hidden_size, output_size)
inputs = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
expected = np.array([[0], [1], [1], [0]])
nn.train(inputs, expected, 10000, 0.1)
(二)BP神经网络的优缺点
- 优点:
- 强大的非线性映射能力,可以学习复杂的数据模式。
- 对噪声数据有一定的鲁棒性。
- 理论基础扎实,易于实现和理解。
- 缺点:
- 训练速度可能较慢,尤其是对于大规模数据和复杂网络结构。
- 容易出现过拟合,特别是当训练数据较少时。
- 对于超参数(如隐藏层的数量和节点数)的选择较为敏感。
二、数据增强技术
(一)数据增强的重要性
数据增强技术旨在通过对原始数据进行变换,生成新的训练样本,从而增加训练数据的多样性,提高模型的泛化能力。这对于解决数据不足和防止过拟合问题至关重要。
(二)常见的数据增强方法
1. 图像数据增强
对于图像数据,常见的数据增强技术包括:
- 旋转:将图像按一定角度旋转。
- 翻转:水平或垂直翻转图像。
- 裁剪:随机裁剪图像的一部分。
- 缩放:对图像进行放大或缩小。
- 添加噪声:在图像中添加随机噪声。
以下是使用 PIL
和 numpy
进行图像数据增强的代码示例:
from PIL import Image
import numpy as np
import random
def rotate_image(image, angle):
pil_image = Image.fromarray(image)
rotated_image = pil_image.rotate(angle)
return np.array(rotated_image)
def flip_image(image, direction):
pil_image = Image.fromarray(image)
if direction == 'horizontal':
flipped_image = pil_image.transpose(Image.FLIP_LEFT_RIGHT)
elif direction == 'vertical':
flipped_image = pil_image.transpose(Image.FLIP_TOP_BOTTOM)
return np.array(flipped_image)
def crop_image(image, crop_size):
h, w = image.shape[:2]
start_h = random.randint(0, h - crop_size)
start_w = random.randint(0, w - crop_size)
cropped_image = image[start_h:start_h + crop_size, start_w:start_w + crop_size]
return cropped_image
def add_noise(image, mean=0, std=10):
noise = np.random.normal(mean, std, image.shape).astype(np.uint8)
noisy_image = np.clip(image + noise, 0, 255)
return noisy_image
# 示例使用
image = np.random.randint(0, 256, (100, 100, 3), dtype=np.uint8)
rotated = rotate_image(image, 45)
flipped = flip_image(image, 'horizontal')
cropped = crop_image(image, 80)
noisy = add_noise(image)
2. 文本数据增强
对于文本数据,可以采用:
- 同义词替换:用同义词替换句子中的某些词。
- 随机插入:在句子中随机插入一些词。
- 随机删除:随机删除句子中的一些词。
- 打乱顺序:打乱句子中词的顺序。
以下是一个简单的文本数据增强示例(使用 nltk
库):
import nltk
from nltk.corpus import wordnet
import random
def get_synonyms(word):
synonyms = []
for syn in wordnet.synsets(word):
for lemma in syn.lemmas():
synonyms.append(lemma.name())
if synonyms:
return random.choice(synonyms)
return word
def synonym_replacement(sentence, n=1):
words = sentence.split()
new_words = words.copy()
random_word_list = list(set([word for word in words]))
random.shuffle(random_word_list)
num_replaced = 0
for random_word in random_word_list:
synonym = get_synonyms(random_word)
if synonym:
new_words = [synonym if word == random_word else word for word in new_words]
num_replaced += 1
if num_replaced >= n:
break
return ' '.join(new_words)
def random_insertion(sentence, n=1):
words = sentence.split()
for _ in range(n):
new_word = random.choice(words)
random_index = random.randint(0, len(words))
words.insert(random_index, new_word)
return ' '.join(words)
def random_deletion(sentence, p=0.5):
words = sentence.split()
if len(words) == 1:
return words[0]
new_words = [word for word in words if random.uniform(0, 1) > p]
if len(new_words) == 0:
return random.choice(words)
return ' '.join(new_words)
def random_swap(sentence, n=1):
words = sentence.split()
for _ in range(n):
idx1 = random.randint(0, len(words) - 1)
idx2 = random.randint(0, len(words) - 1)
words[idx1], words[idx2] = words[idx2], words[idx1]
return ' '.join(words)
# 示例使用
sentence = "This is a sample sentence"
augmented_sentence1 = synonym_replacement(sentence)
augmented_sentence2 = random_insertion(sentence)
augmented_sentence3 = random_deletion(sentence)
augmented_sentence4 = random_swap(sentence)
3. 时间序列数据增强
对于时间序列数据,可以采用:
- 时间扭曲:拉伸或压缩时间序列。
- 幅度缩放:对时间序列的幅度进行缩放。
- 添加噪声:添加高斯噪声等。
以下是一个简单的时间序列数据增强示例:
import numpy as np
def time_warp(series, factor=0.2):
length = len(series)
warp_factor = np.random.uniform(-factor, factor) + 1
new_length = int(length * warp_factor)
indices = np.linspace(0, length - 1, new_length)
indices = np.clip(indices, 0, length - 1).astype(int)
return series[indices]
def amplitude_scale(series, factor=0.2):
scale_factor = np.random.uniform(1 - factor, 1 + factor)
return series * scale_factor
def add_gaussian_noise(series, mean=0, std=0.1):
noise = np.random.normal(mean, std, len(series))
return series + noise
# 示例使用
time_series = np.sin(np.linspace(0, 2 * np.pi, 100))
warped_series = time_warp(time_series)
scaled_series = amplitude_scale(time_series)
noisy_series = add_gaussian_noise(time_series)
三、BP神经网络与数据增强技术的结合应用
(一)图像分类任务
在图像分类任务中,将数据增强技术应用于训练集,再使用 BP 神经网络进行训练。以下是使用 Keras
框架的示例:
from keras.models import Sequential
from keras.layers import Dense, Flatten
from keras.utils import to_categorical
from keras.preprocessing.image import ImageDataGenerator
# 假设数据已经加载,X_train 是训练图像数据,y_train 是训练标签,X_test 是测试图像数据,y_test 是测试标签
# 数据增强生成器
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
horizontal_flip=True,
vertical_flip=True,
zoom_range=0.2,
fill_mode='nearest'
)
# 构建 BP 神经网络模型
model = Sequential([
Flatten(input_shape=(28, 28, 3)), # 假设图像大小为 28x28x3
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 使用数据增强生成器训练模型
model.fit_generator(datagen.flow(X_train, to_categorical(y_train), epochs=10, validation_data=(X_test, to_categorical(y_test)))
(二)文本分类任务
在文本分类任务中,结合文本数据增强技术:
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
from keras.models import Sequential
from keras.layers import Embedding, LSTM, Dense
# 假设文本数据存储在 texts 中,标签存储在 labels 中
tokenizer = Tokenizer(num_words=10000)
tokenizer.fit_on_texts(texts)
sequences = tokenizer.texts_to_sequences(texts)
word_index = tokenizer.word_index
maxlen = 100
data = pad_sequences(sequences, maxlen=maxlen)
def augment_text_data(texts, labels, n_augment=2):
augmented_texts = []
augmented_labels = []
for text, label in zip(texts, labels):
augmented_texts.append(text)
augmented_labels.append(label)
for _ in range(n_augment):
augmented_text = random_insertion(text)
augmented_texts.append(augmented_text)
augmented_labels.append(label)
return augmented_texts, augmented_labels
augmented_texts, augmented_labels = augment_text_data(texts, labels)
augmented_sequences = tokenizer.texts_to_sequences(augmented_texts)
augmented_data = pad_sequences(augmented_sequences, maxlen=maxlen)
# 构建 BP 神经网络模型,这里使用 LSTM 作为示例
model = Sequential([
Embedding(10000, 128),
LSTM(128),
Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(np.array(augmented_data), np.array(augmented_labels), epochs=10)
(三)时间序列预测任务
对于时间序列预测,结合时间序列数据增强技术:
import numpy as np
from keras.models import Sequential
from keras.layers import LSTM, Dense
def augment_time_series_data(series, labels, n_augment=2):
augmented_series = []
augmented_labels = []
for s, label in zip(series, labels):
augmented_series.append(s)
augmented_labels.append(label)
for _ in range(n_augment):
warped = time_warp(s)
scaled = amplitude_scale(warped)
noisy = add_gaussian_noise(scaled)
augmented_series.append(noisy)
augmented_labels.append(label)
return np.array(augmented_series), np.array(augmented_labels)
# 假设时间序列数据存储在 time_series 中,标签存储在 time_labels 中
augmented_series, augmented_labels = augment_time_series_data(time_series, time_labels)
# 重塑数据以适应 LSTM 输入
X = np.reshape(augmented_series, (augmented_series.shape[0], augmented_series.shape[1], 1))
# 构建 BP 神经网络模型,这里使用 LSTM 作为示例
model = Sequential([
LSTM(50, activation='relu', input_shape=(X.shape[1], X.shape[2])),
Dense(1)
])
model.compile(optimizer='adam', loss='mse')
model.fit(X, augmented_labels, epochs=10)
四、实验结果与分析
(一)实验设置
为了验证 BP 神经网络结合数据增强技术的有效性,我们在上述三个任务中分别设置对照组(仅使用原始数据训练的 BP 神经网络)和实验组(使用增强数据训练的 BP 神经网络),并对比它们的性能。
(二)实验结果
在图像分类任务中,使用数据增强的模型在测试集上的准确率明显高于未使用数据增强的模型,尤其在训练数据量较少时,提升更为显著。对于文本分类和时间序列预测任务,也得到了类似的结果,模型的泛化能力得到了显著提高,过拟合现象得到缓解。
五、结论
BP 神经网络与数据增强技术的结合在多个领域展现出强大的性能优势。数据增强技术通过增加数据的多样性,有效地帮助 BP 神经网络克服了过拟合问题,提高了模型在不同任务中的泛化能力。然而,数据增强的效果也取决于增强方法的选择和超参数的调整,需要根据具体的任务和数据类型进行合理的设计和优化。在实际应用中,通过不断地实验和调整,可以使两者的结合发挥最大的效能,为不同领域的分类、预测和分析任务提供更强大的解决方案。
以上代码示例仅为演示目的,在实际应用中可能需要根据具体的数据特点、任务要求和性能指标进行进一步的优化和调整。同时,不同的数据增强技术可以组合使用,以达到更好的效果,不同的深度学习框架和工具也可以根据需要进行选择和切换。
更多推荐
所有评论(0)