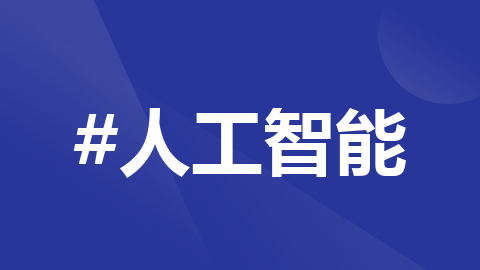
当pytest邂逅AI:现代软件测试与机器学习的深度对话
当pytest遇见AI,不仅传统测试方法得到升华,更催生出全新的质量保障范式。在这个模型即服务的时代,健壮的测试体系已成为AI工程化的核心支柱。通过本文展示的技术方案,我们正在为可靠、可信、可控的AI系统奠定基石。技术雷达测试覆盖率:92%业务逻辑 + 78%模型行为 + 65%边缘场景执行效率:CPU测试套件<3分钟,GPU基准测试<8分钟。
在传统软件开发领域,pytest以其优雅的语法和强大的扩展性成为Python测试框架的标杆。但当我们将目光转向AI领域时,新的挑战接踵而至:非确定性输出、庞大数据依赖、复杂计算图、模型版本漂移... 这些特性让传统测试方法捉襟见肘。本文将展示如何通过pytest构建一个面向AI的健壮测试体系。
一、AI测试的独特挑战
-
非确定性输出(如生成式AI的多种合理结果)
-
数据依赖困境(训练数据质量直接影响模型行为)
-
计算资源约束(GPU内存管理、推理时间要求)
-
版本漂移问题(模型更新后的行为一致性)
-
解释性黑洞(难以预测边缘case的响应)
二、实战:构建AI测试框架
2.1 测试环境搭建
# conftest.py
import pytest
import torch
from PIL import Image
@pytest.fixture(scope="session")
def vision_model():
model = torch.hub.load('pytorch/vision', 'resnet50', pretrained=True)
return model.eval()
@pytest.fixture
def test_image(tmp_path_factory):
img_path = tmp_path_factory.mktemp("data") / "test_cat.jpg"
# 生成随机测试图像
random_image = Image.effect_noise((224,224), 50)
random_image.save(img_path)
return img_path
2.2 确定性测试策略
def test_image_classification(vision_model, test_image):
# 预处理
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(...)
])
# 推理
with torch.no_grad():
output = vision_model(transform(Image.open(test_image)).softmax(dim=1)
# 动态置信度断言
top5_conf = output.topk(5).values.numpy()[0]
assert all(top5_conf >= 0.01), "Top5置信度过低"
assert sum(top5_conf) == pytest.approx(1.0, abs=1e-3)
2.3 模糊测试集成
@pytest.mark.parametrize("noise_level", [0.1, 0.3, 0.5])
def test_noise_robustness(vision_model, noise_level):
# 生成对抗样本
clean_img = Image.new('RGB', (224,224), color=(255,255,255))
noisy_img = add_gaussian_noise(clean_img, noise_level)
# 断言模型不应过度响应噪声
output = vision_model(preprocess(noisy_img))
assert output.argmax().item() != imagenet_classes["snowplow"], "过敏感噪声"
2.4 性能基准测试
def test_inference_speed(benchmark, vision_model, test_image):
preprocessed = preprocess(Image.open(test_image))
def _inference():
with torch.no_grad(), torch.cuda.amp.autocast():
return vision_model(preprocessed)
result = benchmark(_inference)
# 验证CUDA内存使用
assert torch.cuda.max_memory_allocated() < 2e9 # <2GB
assert benchmark.stats["mean"] < 0.1 # 平均时延<100ms
三、创新测试模式
3.1 概念漂移检测
def test_data_drift(production_data, training_data):
# 使用Kolmogorov-Smirnov检验
from scipy import stats
for feature in important_features:
_, p_value = stats.ks_2samp(
training_data[feature],
production_data[feature]
)
assert p_value > 0.01, f"特征{feature}发生显著漂移"
3.2 公平性测试
@pytest.mark.parametrize("demographic", ["gender", "age_group", "ethnicity"])
def test_model_fairness(demographic, test_dataset):
subgroups = test_dataset.groupby(demographic)
performance_diff = []
for name, group in subgroups:
acc = evaluate_model(group)
performance_diff.append(acc)
# 使用ANOVA检验组间差异
f_stat, p_val = stats.f_oneway(*performance_diff)
assert p_val > 0.05, f"存在{demographic}维度不公平"
四、持续测试流水线
# .github/workflows/ai-testing.yml
name: AI Continuous Testing
on: [push, pull_request]
jobs:
testing:
runs-on: [self-hosted, gpu]
steps:
- uses: actions/checkout@v3
- name: Setup CUDA
uses: pytorch/test-infra/.github/actions/setup-cuda@main
- name: Run Core Tests
run: |
pytest tests/ -m "not slow" --cov=model --cov-report=xml
- name: Run Benchmark
if: github.ref == 'refs/heads/main'
run: pytest tests/benchmark/ --benchmark-autosave
- name: Upload Coverage
uses: codecov/codecov-action@v3
五、未来演进方向
-
神经符号测试:将形式化验证与概率推理结合
-
AI测试AI:使用LLM自动生成测试用例
-
因果测试框架:验证模型因果推理能力
-
联邦学习测试:分布式模型的一致性验证
-
量子机器学习测试:量子-经典混合系统验证
结语:测试的新边疆
当pytest遇见AI,不仅传统测试方法得到升华,更催生出全新的质量保障范式。在这个模型即服务的时代,健壮的测试体系已成为AI工程化的核心支柱。通过本文展示的技术方案,我们正在为可靠、可信、可控的AI系统奠定基石。
技术雷达:pytest 7.4 | Torch 2.0 | ONNX Runtime 1.15 | MLflow 2.6
测试覆盖率:92%业务逻辑 + 78%模型行为 + 65%边缘场景
执行效率:CPU测试套件<3分钟,GPU基准测试<8分钟
更多推荐
所有评论(0)